문자열
char str[6];
str[0] = 'h';
str[1] = 'e';
str[2] = 'l';
str[3] = 'l';
str[4] = 'o';
str[5] = '\0';
이런식인 경우
문자열에 끝에 '\0' 값을 넣어주지 않으면 제대로 동작하지 않는다.
문자열이 끝났는지 아닌지 알기 위해서 '\0' 써야한다.
단, char str[] = "hello";
char *str ="hello";
과 같이 표현하는 경우 '\0'를 문자열 끝에 넣어주지 않아도 된다.
하지만 char *str ="hello"; 로 표현하는 경우 문자열 수정이 불가능하다하여 string literal이라고 한다.
엄밀하게 말하면 문자열 배열이 아닌 string literal이다.
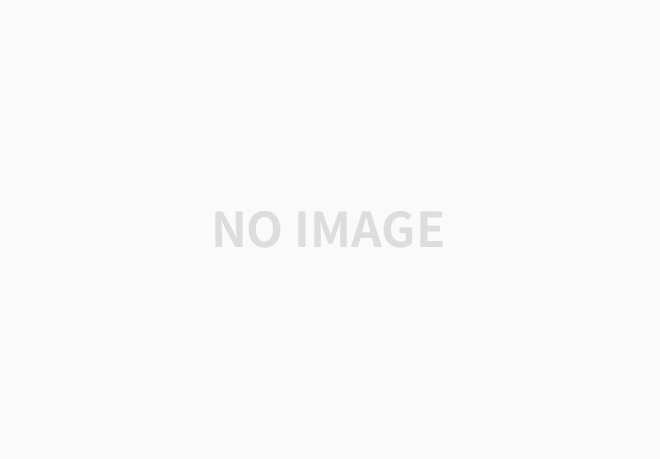
사실 char str[] = "hello" 이것과 char *str ="hello" 이것의 차이가 *로 표현하였느냐 차이인데 둘이 다르다는 점이 잘 와닿지는 않다.
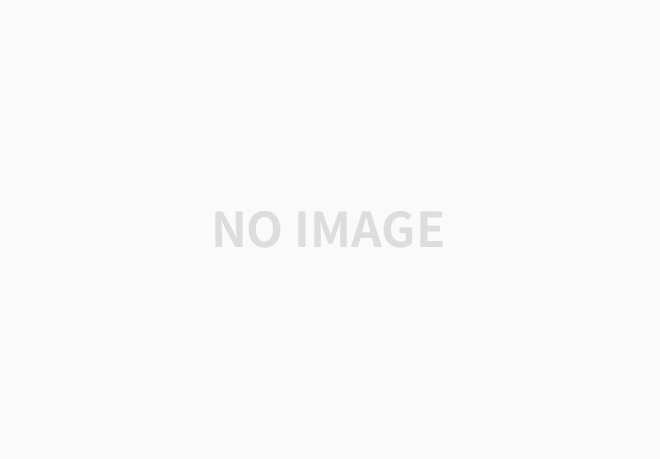
위와 같이 배열 원소 하나하나가 포인터 변수로 쓰이기 위해서는 아래와 같이 해야한다.
#define BUFFER_SIZE=100
int main() {
char *words[100];
char buffer[BUFFER_SIZE];
int n = 0; // number of strings
while( scanf("%s", buffer) != EOF ) {
words[n] = strdup(buffer); // strcpy(str2, str1)
n++;
// buffer로 하면 될거 같지만 buffer로만하면 buffer자체도 포인터 변수이므로 맨 마지막에 입력한 단어의 값만 저장된다.
}
strdup는 아래와 같다.
char strdup(char *s) {
char *p;
p = (char *)malloc(strlen(s)+1); //+1 는 \0를 위해 사용
if ( p != NULL)
strcpy(p, s)
return p;
}
파일로부터 읽기
#include <stdio.h>
void main() {
FILE *fp = fopen("input.txt, "r");
char buffer[100];
while(fscanf(fp, "%s", buffer) != EOF)
printf("%s", buffer);
fclose(fp);
파일 읽고 쓰기
#include <stdio.h>
void main() {
FILE *in_fp = fopen("input.txt, "r");
FILE *out_fp = fopen("input.txt, "w");
char buffer[100];
while(fscanf(in_fp , "%s", buffer) != EOF)
fprintf(out_fp , "%s", buffer);
fclose(in_fp );
fclose(out_fp );
쓴거부터 닫고 읽기 표시된 것을 마지막에 닫는게 자연스럽지 않나 싶었지만 그렇지 않으며
파일 연거부터 닫는다.
'CS > 자료구조' 카테고리의 다른 글
[인프런|6,7,8강] C로 배우는 자료구조 | 전화번호부 v3.0 (0) | 2020.11.15 |
---|---|
[인프런|5강] C로 배우는 자료구조 | 전화번호부 v2.0 (0) | 2020.11.14 |
[인프런|4강] C로 배우는 자료구조 | 전화번호부 v1.0 (0) | 2020.11.13 |
[인프런|3강] C로 배우는 자료구조 | 문자열 연습문제 (0) | 2020.11.11 |
[인프런|1강] C로 배우는 자료구조 | C언어 기초 문법 리뷰 (0) | 2020.11.09 |